JavaScript step
Run custom JavaScript to manipulate variables or control logic.
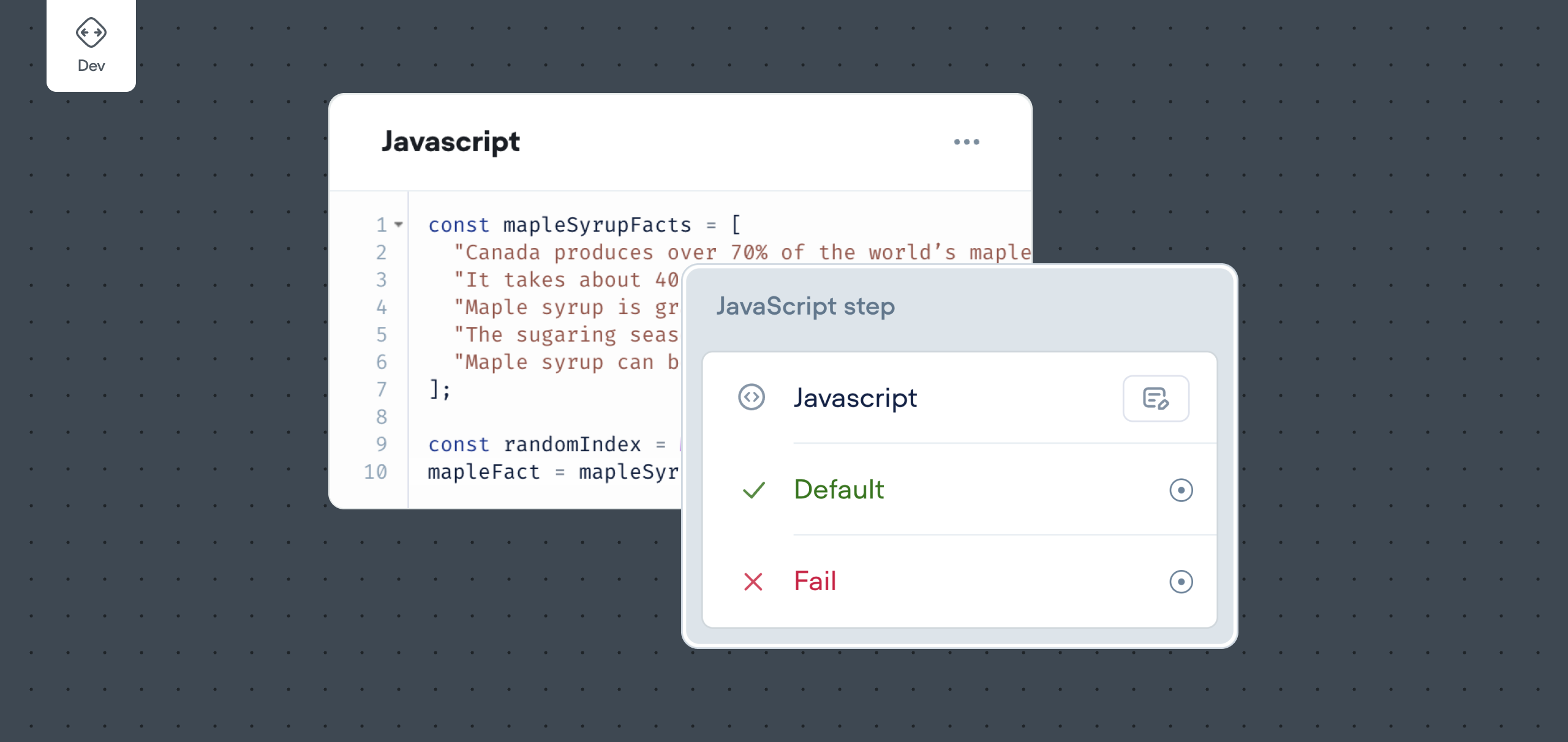
The JavaScript step lets you write and execute short JavaScript snippets (ES6 on V8) directly in your agent. It’s useful for logic that doesn’t require an external API call or reusable function like parsing JSON, modifying variables, or adding conditional paths.
This step runs once when reached and evaluates immediately. If your use case involves longer or reusable code, we recommend using a Function instead.
Basic usage
To use the JavaScript step, drag it onto the canvas and click into the step to open the code editor.
All of the agent's variables are automatically available for use—no need to reference them with curly braces. You can update variable values by reassigning them directly.
Here's an example of a JavaScript step that increments the score
variable by 1:
Paths
The JavaScript step supports paths, which allow you to route your agent based on the value that is returned by the JavaScript step. There are two ways to use paths: with the standard paths, or using custom paths.
Standard paths
The code step has two standard paths: Default
and Fail
. The Default path will be followed if your code is successfully run, and the Fail path will be followed if your code fails to run or has an error during its execution.
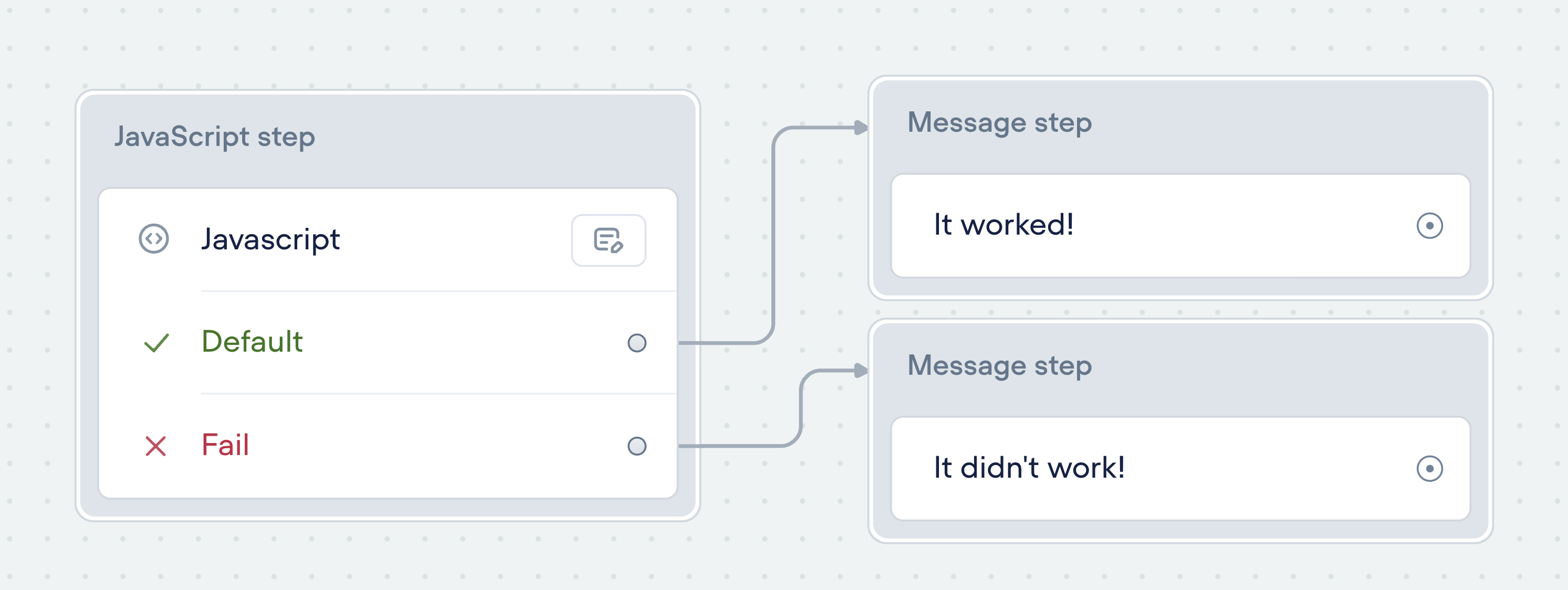
Custom paths
You can add up to 10 custom paths to route users based on your logic. Each path must be named. Use a return [path_name]
statement in your code to route the agent to that path. If no path is returned, the flow continues through the Default
path.
Here's example with two custom paths: victory
, defeat
. If the score
variable is above 100
, victory
is returned. If score
is less than 0
, then defeat
is returned. Otherwise, the Default
path is followed.
if (score > 100) {
return "victory"
} else if (score < 0) {
return "defeat"
}
// otherwise it will go down the Default path here
Limitations
Some constraints to consider when using the JavaScript step include:
- JavaScript steps cannot be used to make requests to external servers, so cannot be used to call external APIs. Alternatively, you can use the API Step to pull or push data between your Voiceflow agent and a third-party system, or functions.
- JavaScript steps do not currently support importing modules.
- JavaScript steps cannot be used to create new variables. Any variables that you want to use after the JavaScript step is executed must already exist before being referenced in the JavaScript step.
Debugging
Common error: TypeError when testing the JavaScript step
In the Creator tool, if a variable hasn’t been set before the step runs, it defaults to 0
. This can lead to a TypeError
if your code expects a string or other type.
For example, this will fail if username hasn’t been set:
username.toLowerCase();
To avoid this, always check for the variable first:
if (username) {
username = username.toLowerCase();
}
Updated 14 days ago