Custom forms and extensions
Render custom widgets like file upload, date pickers, and more!
Voiceflow's extensions feature lets you add advanced functionality to your web chat experience. Extensions allow your assistant to render custom widgets or trigger custom effects directly on your website.
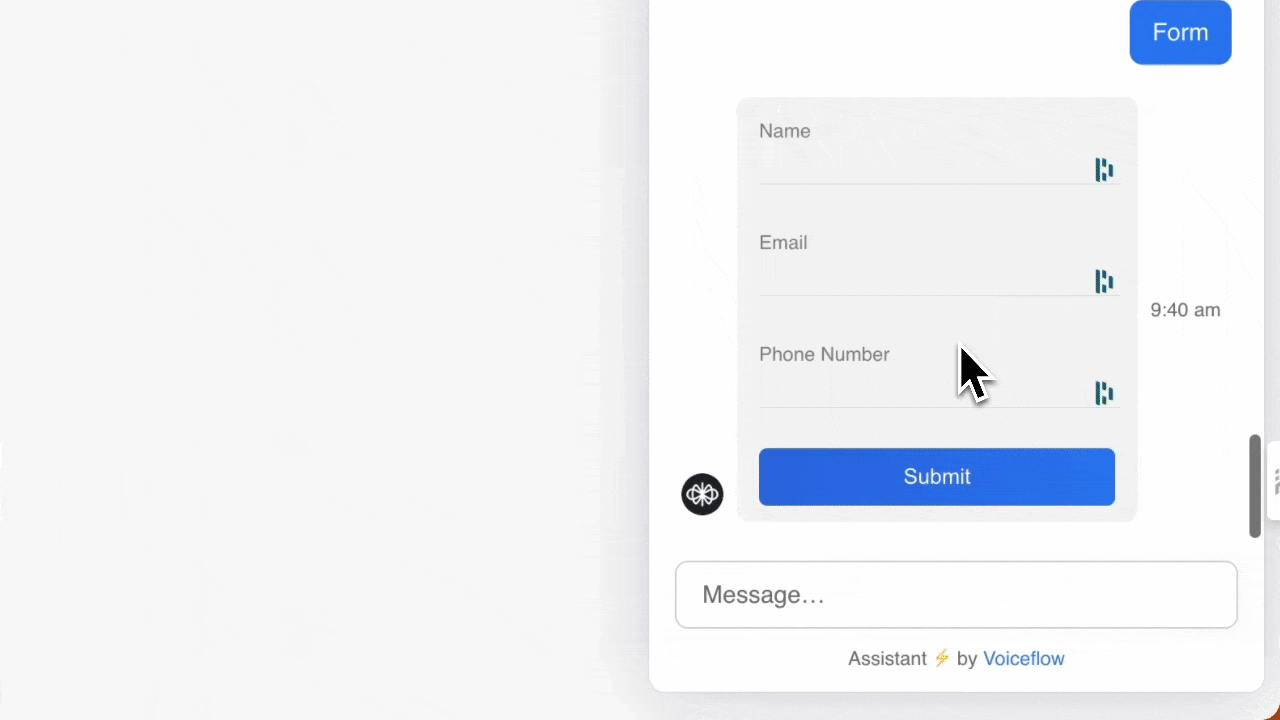
There are two types of extensions:
- Response extensions: these render interactive widgets inside the web chat window like file uploads, calendar pickers, or payment modals.
- Effect extensions: these don’t render a UI element but can trigger changes elsewhere on your site, such as updating a status icon, deep-linking a user, or running custom scripts.
Want to jump into code? Find examples on GitHub.
How extensions work
Extensions are triggered by a Custom action step or a Function step in your Voiceflow assistant. You define them in your site’s code and register them in the web chat snippet.
An extension follows the pattern below:
Response Extension
interface ResponseExtension {
name: string,
type: "response",
match: (args: { trace: Trace }) => boolean,
render?: (args: { trace: Trace, element: HTMLDivElement }) => (() => void) | void
}
Effects Extension
interface EffectExtension {
name: string,
type: "effect",
match: (args: { trace: Trace }) => boolean,
effect?: (args: { trace: Trace }) => Promise<void> | void
}
Registering extensions in the web chat widget
Once you've defined your extensions, register them using the assistant.extensions
property in your chat.load()
script:
window.voiceflow.chat.load({
verify: { projectID: 'YOUR_PROJECT_ID' },
url: 'https://general-runtime.voiceflow.com',
versionID: 'production',
assistant: {
extensions: [extension1, extension2, extension3] // your extension's name goes here
}
});
There’s no limit to the number of extensions you can include.
Examples
We recommend starting with an example extension before building your own. Our simplest example is a form input extension. Find out how to build it in the doc below.
When you're ready to start experimenting with other types of extensions, visit our sample extensions repo, which has a bunch of different examples for you to try out.
Updated about 1 month ago